2.4. Updating a Key-Value Pair
The Update user interface consists of a labeled selection field (named "key") for choosing the entry to be updated, an input field (named "value") for entering the value (the phone number), and a Save button.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta charset="UTF-8" /> <title>Phone Number Directory</title> </head> <body> <h1>Phone Number Directory</h1> <h2>Update an entry</h2> <form> <p><label>Name: <select name="key" onchange="fillForm()"></select></label></p> <p><label>Phone no.: <input name="value"/></label></p> <p><button type="button" onclick="save()">Save</button></p> </form> <hr/> <nav>...</nav> <script>...</script> </body> </html> |
The following script
element contains the JavaScript code for filling the selection list with option
elements and the code of the functions fillForm
and save
. The function fillForm
is called when a name has been chosen in the selection field. The save
function assigns the updated value from the input field to the selected key in the localStorage
store.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | <script> // get a handle for accessing the form element let formEl = document.forms[0]; // get a handle for accessing the "key" selection field let selectEl = formEl.key; // fill selection list with options for (let key of Object.keys( localStorage)) { let optionEl = document.createElement("option"); optionEl.text = key; selectEl.add( optionEl, null); } // fill form when an entry has been selected function fillForm() { formEl.value.value = localStorage[selectEl.value]; } // save in key-value store function save() { localStorage[selectEl.value] = formEl.value.value; } </script> |
Exercise: Copy and paste the HTML code into a fresh editor window. Don't forget to insert both the content of the nav
element (from a code listing above) and the content of the script
element. Save the resulting user interface page in your example folder as "update.html". Then open the "update.html" file in a browser. You should see a page containing a user interface as in Figure 2-3 below. Choose a name such that the associated phone number is shown. Change this phone number and click the Save button. Then click on Home in the menu for checking if the chosen key-value pair has been updated.
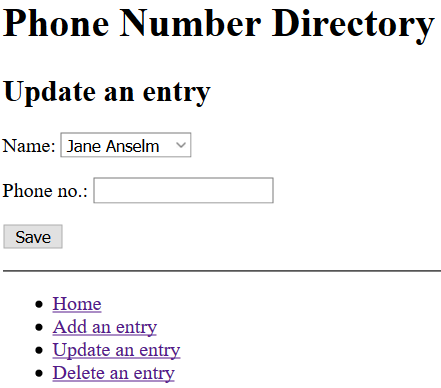